
Multiple threads should not access a PriorityQueue Note that this implementation is not synchronized. Traversal, consider using Arrays.sort(pq.toArray()). The priority queue in any particular order. The Iterator provided in method iterator() is not guaranteed to traverse the elements of Optional methods of the Collection and Iterator interfaces. This class and its iterator implement all of the As elements are added to a priority queue, its capacity It is always at least as large as the queue The queue retrieval operations poll,Ī priority queue is unbounded, but has an internalĬapacity governing the size of an array used to store theĮlements on the queue. Tied for least value, the head is one of those elements - ties areīroken arbitrarily. The head of this queue is the least element
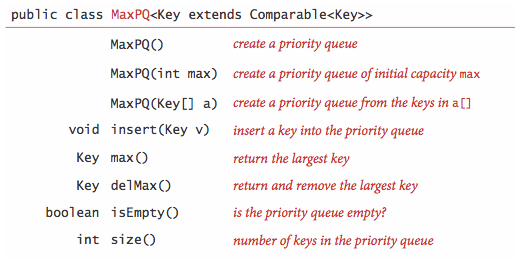
Insertion of non-comparable objects (doing so may result in A priority queue does not permit null elements.Ī priority queue relying on natural ordering also does not permit Provided at queue construction time, depending on which constructor is The elements of the priority queue are ordered according to their An example of a class implementing this interface, Student.java, just passes the work off to unbounded priority queue based on a priority heap. Remember that with the Comparable interface, we use the one.compareTo(other) to decide if one is "less than" (the value is negative), "equal to" (the value is 0), or "greater than" (positive) the other. However, as usual Java chooses different names. This implementation is based on a heap, which we will see later. Java provides a class, although surprisingly no interface for a general priority queue. We will focus on min-priority queues, but they are really the same thing. We can also define a max-priority queue, which replaces the latter operations by maximum and extractMax (and increaseKey if you include it). The job of implementing a priority queue. Operation, which reduces the priority value in a given node, but this complicates (Note - for some applications it is useful to also have a decreaseKey Queue with the smallest key and removes it from the priority extractMin, which returns the element in the priority.Queue with the smallest key, but leaves the element in the minimum, which returns the element in the priority.insert, which inserts an element into the priority.isEmpty, a predicate that tells whether the.The MinPriorityQueue interface supports the following operations: The way that we have defined the interface, the generic type is E extends Comparable - as we saw with BSTs, this lets us rely on a compareTo() method of the elements, to establish the priority order. Here, each element has a value, which we call its key. MinPriorityQueue.java contains the interface for a min-priority queue. Sometimes, the result of an event is to allow some other event, already scheduled, to occur even earlier in this case, the decreaseKey method changes the time that the other event occurs to move it earlier.
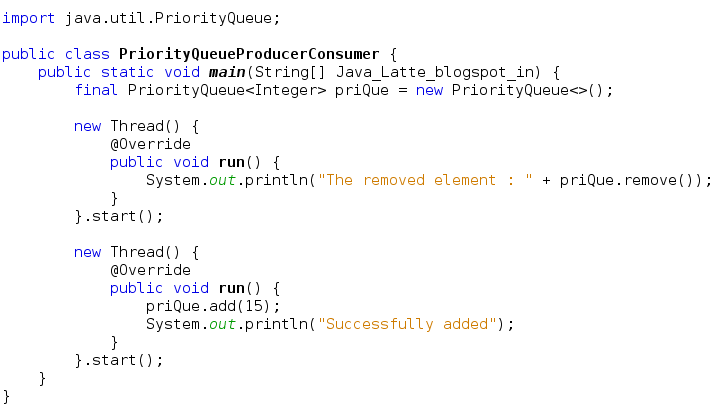
The extractMin method tells us the next event to occur, removing it from the queue so that we don't try to process it more than once. When an event is created, the insert method adds it to the min-priority queue.
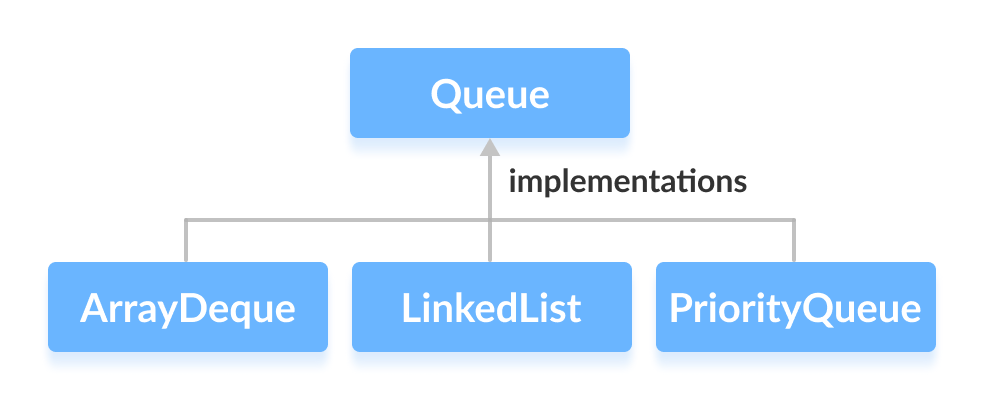
A min-priority queue keeps track of the events that have yet to occur, with the key of an element being the time that its event is to occur. An event can cause another event to occur at some later time. The simulation runs through time, where the time of each occurrence is nondecreasing (i.e., the simulated time either increases or stays the same-it never backs up). Min-priority queues also form the heart of discrete event simulators, which simulate systems in which things happen at various moments in time. And a priority queue gives you an easy way to sort - put everything into the priority queue, then take them out one at a time. They are used in finding shortest paths and other search problems. They come up quite commonly in computer systems (high-priority print jobs, various priority levels of jobs running on a time sharing system. There are hundreds of applications of priority queues. That may seem backwards, but think of "you are our number one priority!" That's better than being their number two or three priority. For a typical priority queue, low priority numbers are removed first.
#Declare priority queue java code
But what if we actually have specific priorities, such that the "most important" thing in should be the first out? That's what priority queues are for.Īll the code files for today: ArrayListMinPriorityQueue.java MinPriorityQueue.java Roster.java SortedArrayListMinPriorityQueue.java Student.javaĪ priority queue is a queue which instead of being FIFO is Best Out.
#Declare priority queue java how to
We saw how to keep things in last-in/first-out or first-in/first-out order.
